MVVM: Separating Logic and Data from UI
The Model-View-ViewModel (MVVM) is a powerful design pattern used for building apps in Swift, particularly when working with SwiftUI. At its core, MVVM emphasizes a strict separation of concerns—keeping the application’s logic and data (the *Model*) separate from the User Interface (the *View*). This separation is critical for ensuring that the app remains maintainable, scalable, and easy to test.
The Model
The *Model* refers to everything that makes up your app’s logic and data. This can range from a simple struct to a complex database, or even machine learning models and APIs. It encapsulates what your app "is"—the data it manages and the rules for manipulating that data.
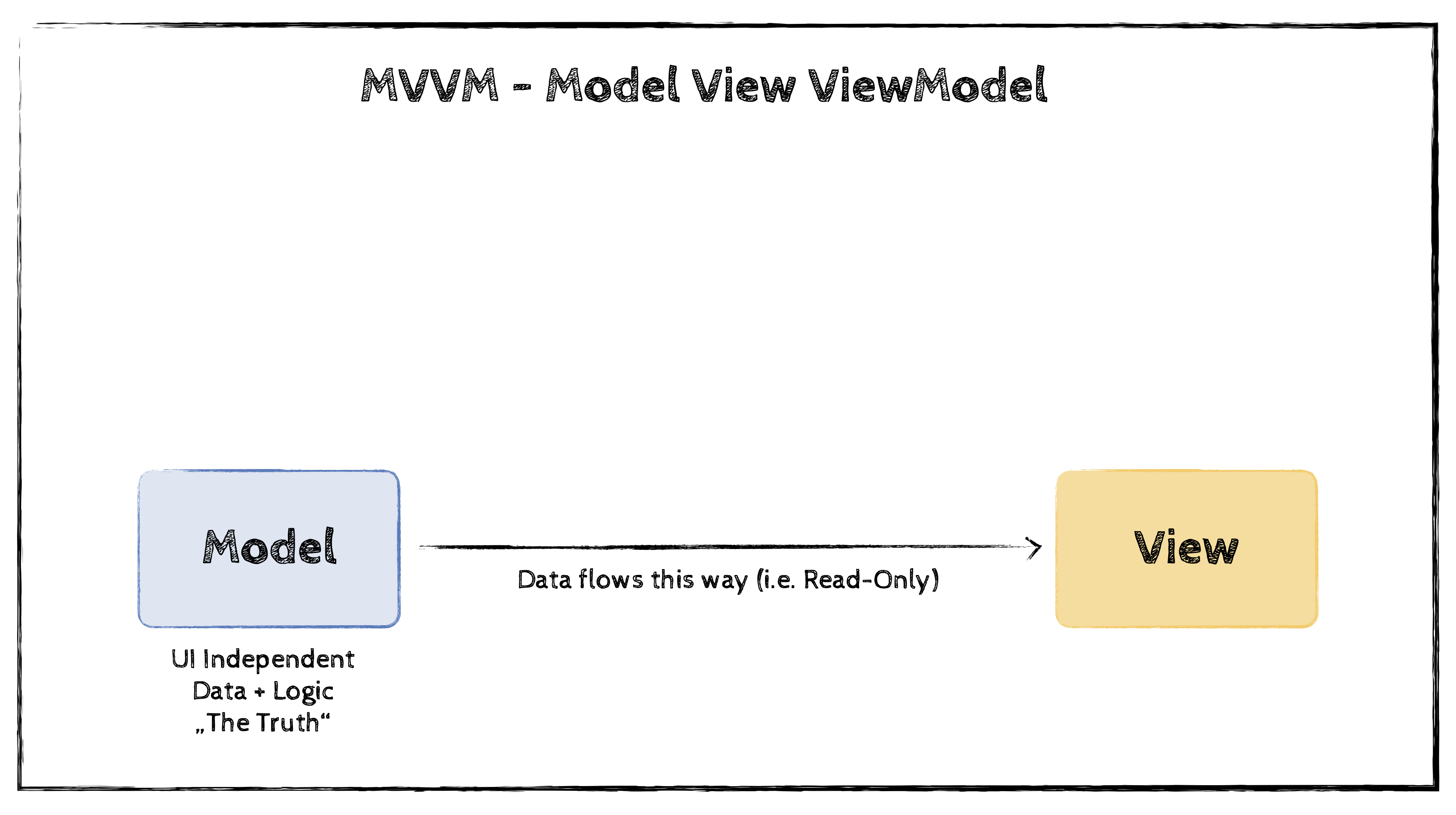
The View
The UI, on the other hand, is just a visual representation of this Model. In other words, the UI is like a “shell” that dynamically reflects changes in the Model, giving users a way to interact with your app’s underlying data. One way to think about it: the UI is merely a *visual manifestation* of the Model. The logic and rules live in the Model; the UI just presents that information.
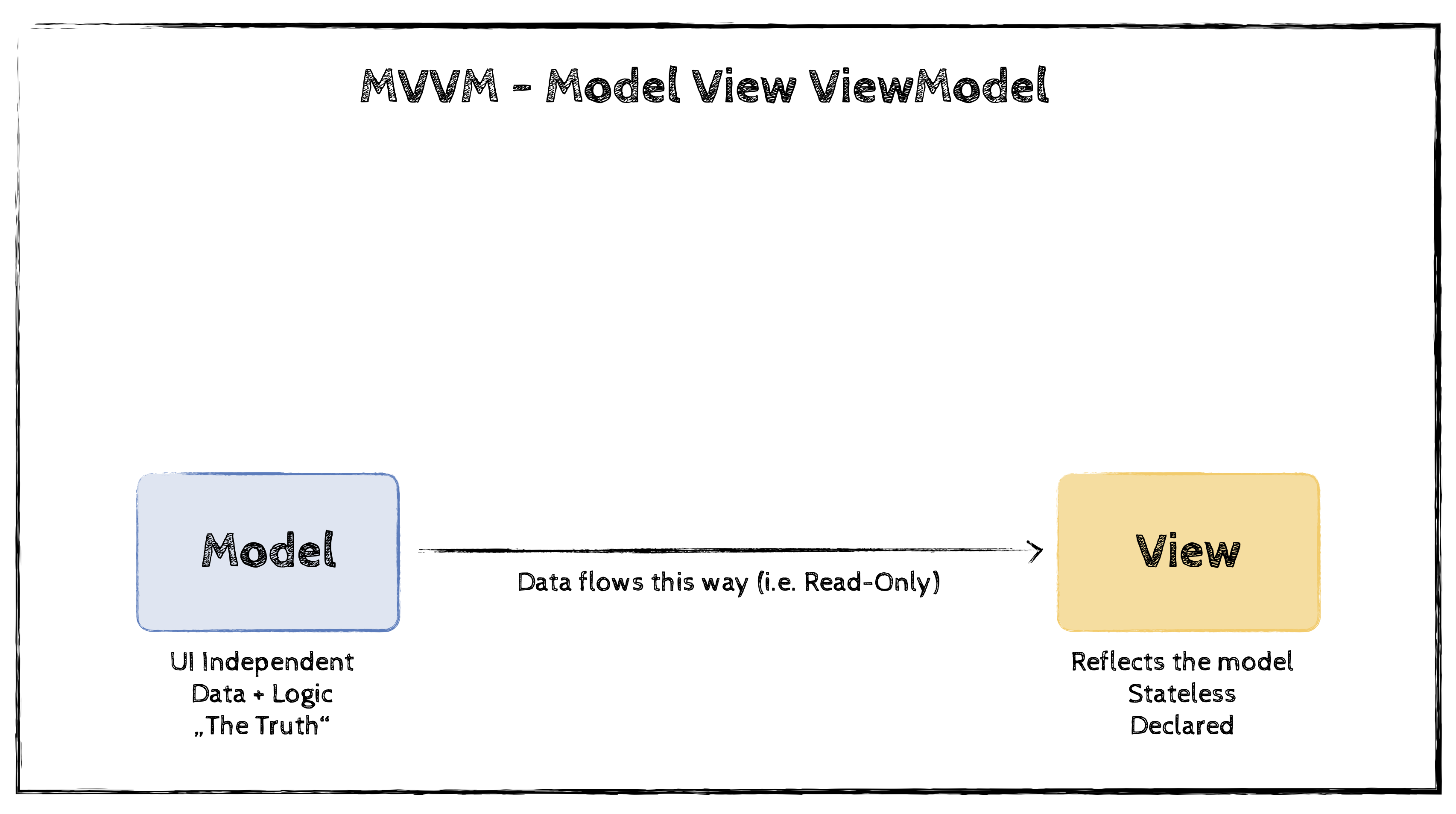
Swift’s Role in MVVM
One of SwiftUI’s core features is its ability to automatically update the UI when the Model changes. Swift provides a built-in infrastructure to ensure that any changes in the Model are reflected in the UI without requiring much manual work. Your job, as the developer, is to give Swift hints about how the Model influences the UI, and Swift will take care of most of the synchronization for you.
The real challenge lies in maintaining a clean separation between the Model and UI, and this is where the ViewModel comes into play.
Connecting the Model and UI: The ViewModel
In MVVM, the *ViewModel* acts as the “gatekeeper” between the Model and the UI. It coordinates how data from the Model is passed to the UI, ensuring that the two interact efficiently and safely. There are three main ways to connect the Model to the UI, but 99% of the time, the ViewModel is the best approach:
- Direct Binding (@State): In some rare cases, you might bind the Model directly to the View using SwiftUI’s `@State`. This approach works if your Model is very simple or short-lived, such as a view that temporarily displays some data and then disappears. However, this minimal separation is generally not recommended for larger, more complex applications.
- Using a ViewModel (MVVM): This is the most common and recommended approach. The ViewModel serves as an intermediary, making sure the UI gets the data it needs from the Model, while keeping the two properly decoupled. The ViewModel can handle all the complexities of data management, such as fetching data from databases or APIs, without burdening the UI with those responsibilities.
- Hybrid Approach: In some cases, the ViewModel might allow limited direct access to the Model through publicly exposed variables. This hybrid approach can be tempting, but it can lead to a tangled codebase over time as the UI starts interacting directly with the Model, which can compromise the flexibility and scalability of your app.
Why Always Choose the ViewModel?
The second approach—using a ViewModel—is the recommended pattern in MVVM because it ensures clean separation and modularity. The ViewModel acts as a gatekeeper that simplifies access to the Model, preventing the UI from making direct calls to data sources like SQL databases or APIs. This makes your UI layer focused purely on presentation, allowing the logic of data retrieval and manipulation to remain isolated in the Model and ViewModel. Even in simple apps, the ViewModel helps ensure scalability and flexibility as the app evolves.
The Role of the ViewModel in MVVM
The ViewModel sits between the Model and the View, binding them together. Its job is twofold:
- Binding and interpreting data: It observes changes in the Model and updates the View accordingly. This is done in a "read-only" fashion, meaning the UI doesn’t directly modify the Model, but simply reacts to changes. If the Model is complex (like a SQL database), the ViewModel may also translate that data into a form that the UI can easily display.
- Handling user intent: The ViewModel also interprets user actions (like tapping a button or selecting an item) and converts these actions into meaningful operations on the Model. For example, if the user selects a card in a game, the ViewModel receives this intent and instructs the Model to update accordingly. Once the Model updates, the ViewModel ensures that the UI reflects the changes.
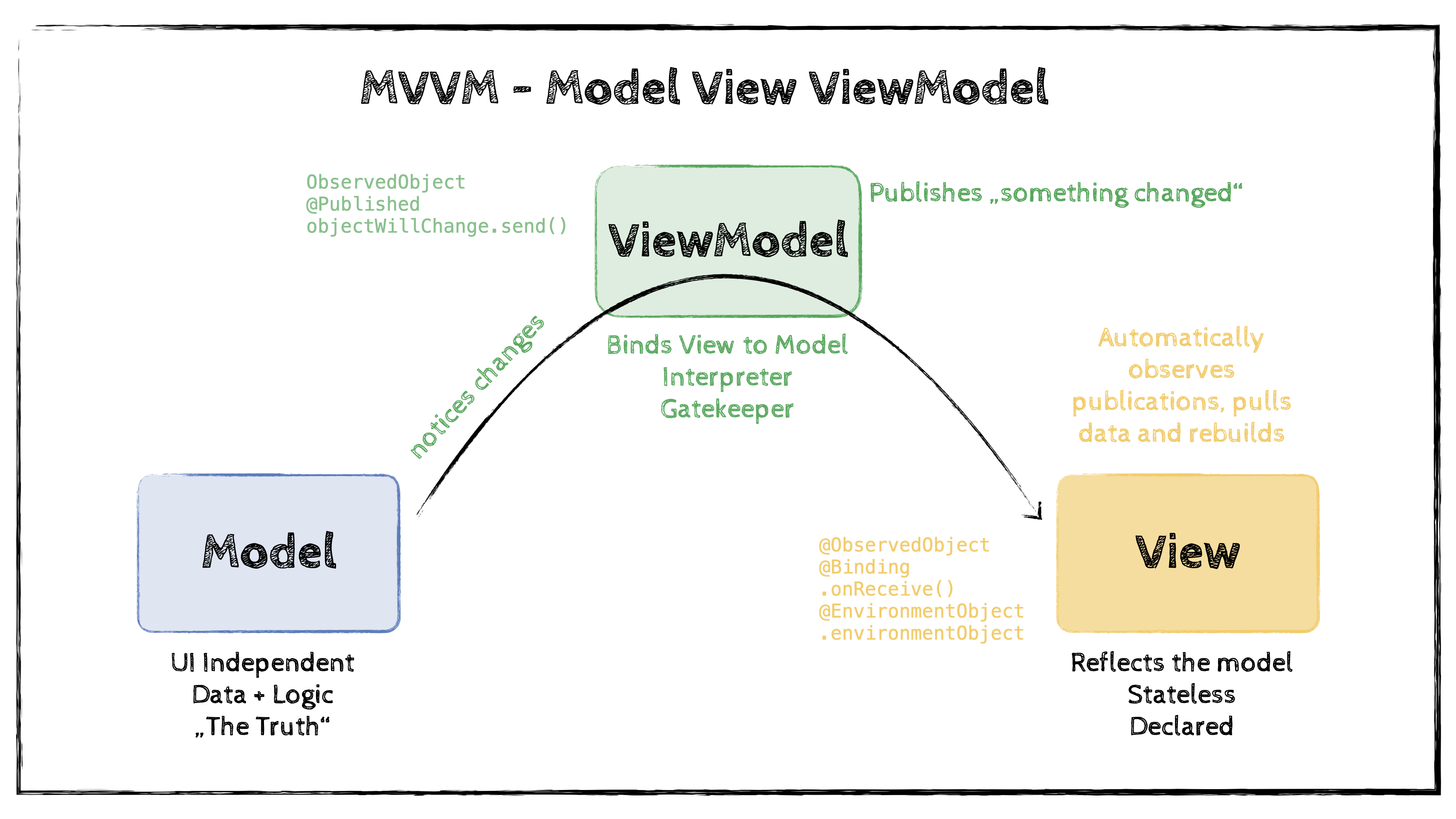
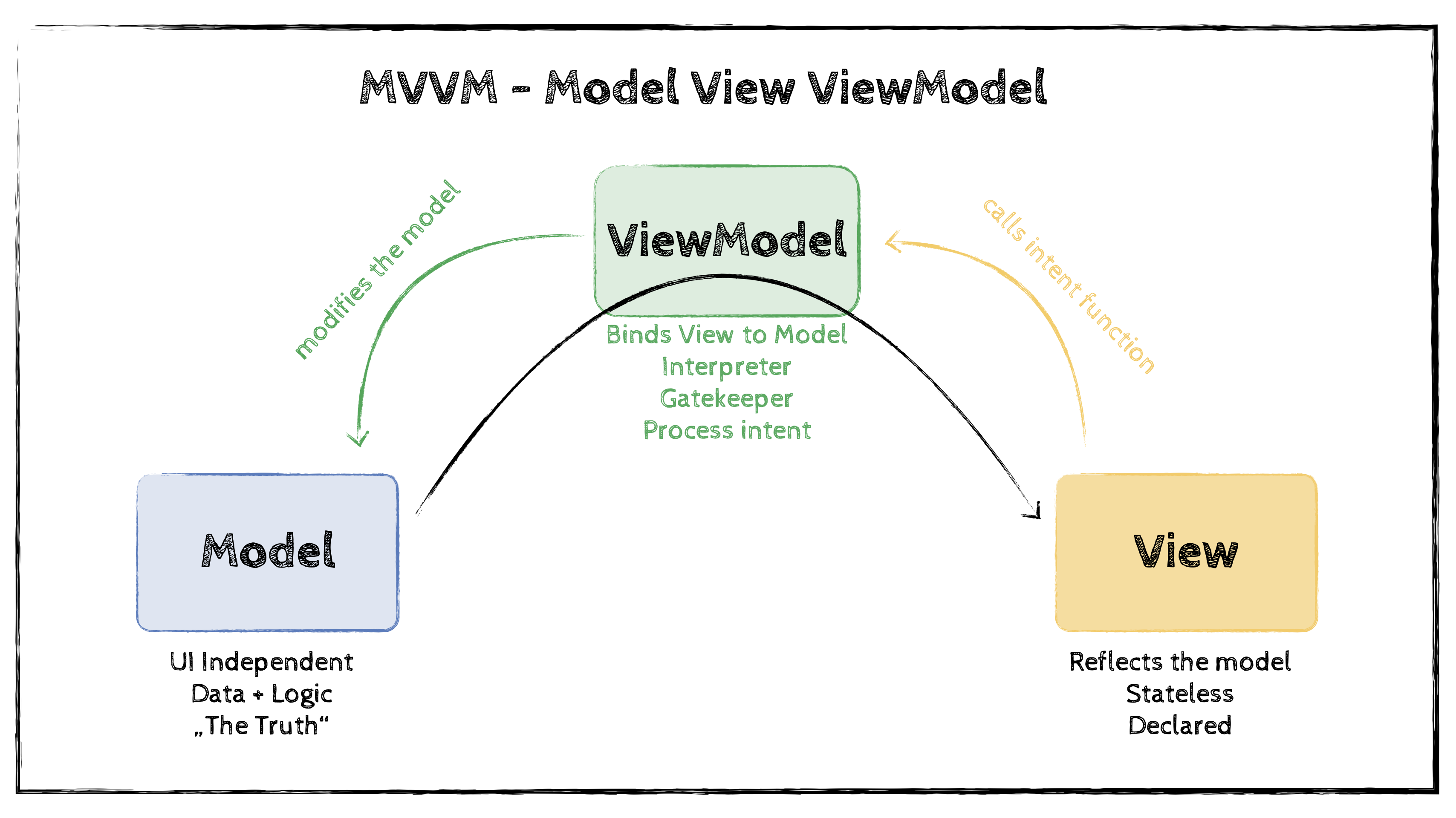
How Data Flows in MVVM
In MVVM, data flows in one direction: from the Model, through the ViewModel, and finally to the UI. The UI remains stateless, simply reacting to changes in the Model. SwiftUI handles the redrawing of only the necessary parts of the UI when the Model changes, optimizing performance.
However, user actions in the UI—like taps or swipes—do need to trigger changes in the Model. This is where "user intent" comes in. The ViewModel translates user interactions into operations on the Model, maintaining a clear separation between the UI and the Model’s logic.
... to be continued!